Using Python's mplfinance and matplotlib libraries to Generate Graphs for Options Trading
Fun with mplfinance, matplotlib, and yfinance for free!
I’ve been working on some python code and the mplfinance, matplotlib, yfinance python libraries to generate the images below. Many of them I have covered called or cash covered puts in which I roll monthly.
import yfinance as yf
import matplotlib.pyplot as plt
import mplfinance as mpf
# Download data
stock_data = yf.download(symbol, period="1y")
# Extract the call/put information for the current stock
cp_type = next(item['CP'] for item in config['stocks'] if item['ticker'] == symbol)
cp_label = "(CALL)" if cp_type == "Call" else "(PUT)"
# Extract the strike price for the current stock
strike_price = next(item['strike_price'] for item in config['stocks'] if item['ticker'] == symbol)
# Calculate the 200-day and 50-day EMA
stock_data['200 EMA'] = stock_data['Close'].ewm(span=200, adjust=False).mean()
stock_data['50 EMA'] = stock_data['Close'].ewm(span=50, adjust=False).mean()
# Create a candlestick chart for the stock (for each day)
fig, ax = plt.subplots(figsize=(16, 6)) # Adjust figure size if needed
# Example plotting:
plt.style.use('ggplot') # Or any style from matplotlib
stock_data['Close'].plot(kind='line', color='blue', title=f"{symbol} Daily Candlestick Chart", ax=ax) # Plot the data with candlesticks
stock_data['200 EMA'].plot(kind='line', color='red', linestyle='--', ax=ax)
stock_data['50 EMA'].plot(kind='line', color='green', linestyle='--', ax=ax)
ax.legend(['Close Price', '200 EMA', '50 EMA']) # Add a legend if needed for clarity
ax.set_title(f"{symbol} Daily Candlestick Chart with 200 and 50 EMA")
ax.set_xlabel('Date')
ax.set_ylabel('Price')
There are a couple types of graphs. The first type are candlestick charts that have the RSI, MACD, and 50/200 Exponential Moving Averages. The second type is a line graph with the EMAs, but also have a yellow line drawn at the strike of my call or put. It’s a nice visualization for me and gives me a quick sense of where the trade is headed. I’d like to combine the 2 into one and move to a weekly, but also add a simple daily line graph to get a sense of the trading day.
Are there indicators you would like to see added that have helped you trade options? Perhaps Average True Range or Bollinger Bands?
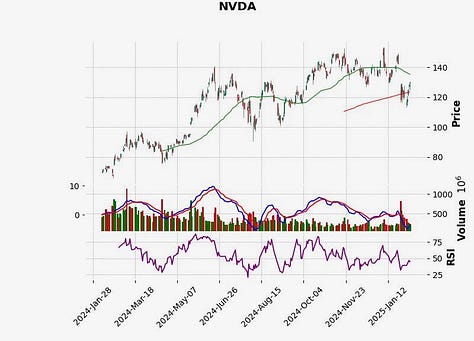
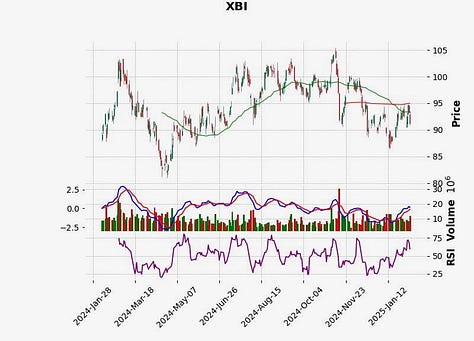
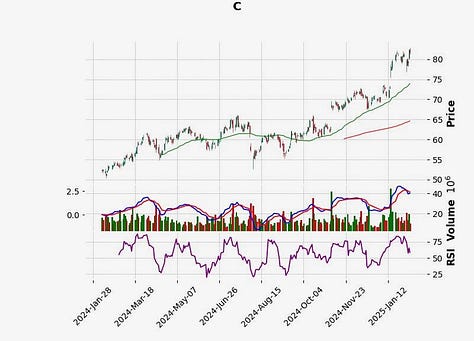
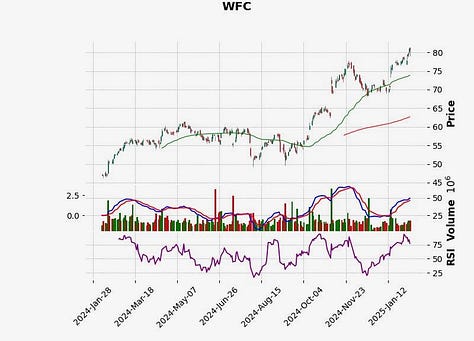
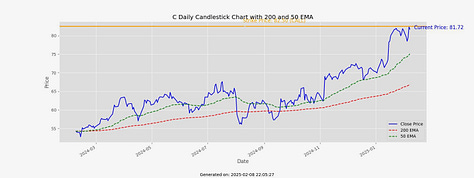
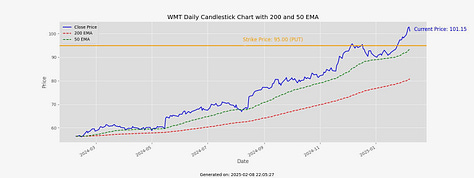
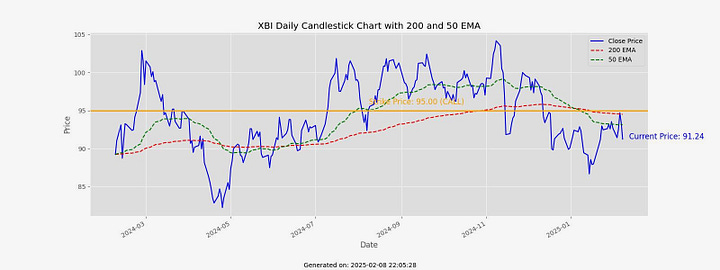
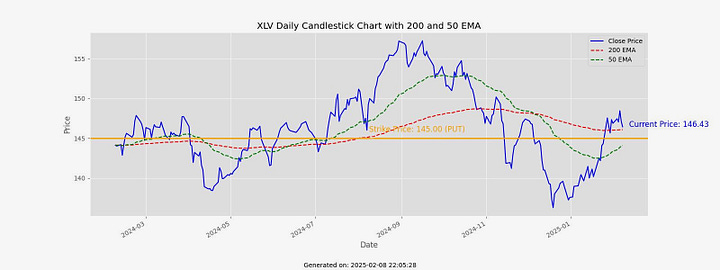
Looking for my trades? Check out Jerry's Active Trades